笔记
单击此处 下载完整的示例代码
Matplotlib 绘图中的文本#
在 Matplotlib 中绘制和处理文本的介绍。
Matplotlib 具有广泛的文本支持,包括对数学表达式的支持、对光栅和矢量输出的 truetype 支持、具有任意旋转的换行符分隔文本以及 Unicode 支持。
因为它将字体直接嵌入到输出文档中,例如,postscript 或 PDF,您在屏幕上看到的就是您在硬拷贝中看到的。
FreeType支持产生非常漂亮的抗锯齿字体,即使在较小的光栅尺寸下也看起来不错。Matplotlib 包含自己的
matplotlib.font_manager
(感谢 Paul Barrett),它实现了跨平台、符合W3C的
字体查找算法。
用户对文本属性(字体大小、字体粗细、文本位置和颜色等)有很大的控制权,并在rc 文件中设置了合理的默认值。重要的是,对于那些对数学或科学图形感兴趣的人,Matplotlib 实现了大量的 TeX 数学符号和命令,支持图形中任何位置的数学表达式。
基本文本命令#
以下命令用于在隐式和显式接口中创建文本(有关权衡的解释,请参见Matplotlib 应用程序接口(API) ):
隐式 API |
显式 API |
描述 |
---|---|---|
在 |
||
在
|
||
|
||
|
||
为
|
||
在 |
||
为 |
所有这些函数都创建并返回一个Text
实例,该实例可以配置各种字体和其他属性。下面的示例显示了所有这些命令的运行情况,更多详细信息将在后面的部分中提供。
import matplotlib
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_subplot()
fig.subplots_adjust(top=0.85)
# Set titles for the figure and the subplot respectively
fig.suptitle('bold figure suptitle', fontsize=14, fontweight='bold')
ax.set_title('axes title')
ax.set_xlabel('xlabel')
ax.set_ylabel('ylabel')
# Set both x- and y-axis limits to [0, 10] instead of default [0, 1]
ax.axis([0, 10, 0, 10])
ax.text(3, 8, 'boxed italics text in data coords', style='italic',
bbox={'facecolor': 'red', 'alpha': 0.5, 'pad': 10})
ax.text(2, 6, r'an equation: $E=mc^2$', fontsize=15)
ax.text(3, 2, 'Unicode: Institut für Festkörperphysik')
ax.text(0.95, 0.01, 'colored text in axes coords',
verticalalignment='bottom', horizontalalignment='right',
transform=ax.transAxes,
color='green', fontsize=15)
ax.plot([2], [1], 'o')
ax.annotate('annotate', xy=(2, 1), xytext=(3, 4),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
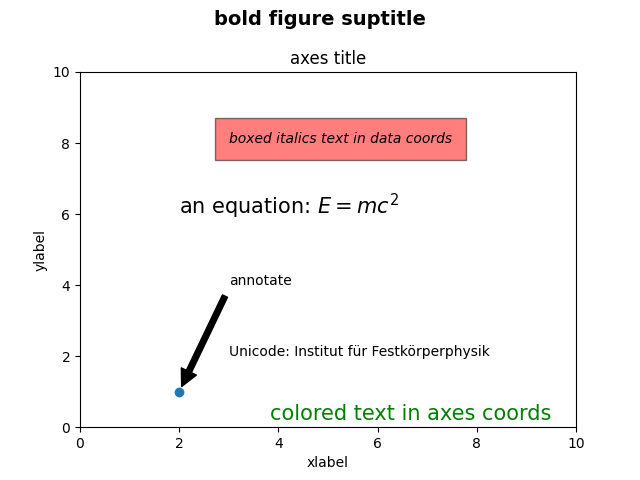
x 轴和 y 轴的标签#
set_xlabel
通过andset_ylabel
方法指定 x 轴和 y 轴的标签很简单
。
import matplotlib.pyplot as plt
import numpy as np
x1 = np.linspace(0.0, 5.0, 100)
y1 = np.cos(2 * np.pi * x1) * np.exp(-x1)
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.15, left=0.2)
ax.plot(x1, y1)
ax.set_xlabel('time [s]')
ax.set_ylabel('Damped oscillation [V]')
plt.show()
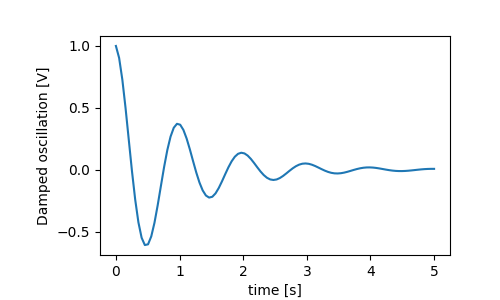
x 和 y 标签会自动放置,以便它们清除 x 和 y 刻度标签。将下图与上图进行比较,注意 y 标签位于上图的左侧。
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.15, left=0.2)
ax.plot(x1, y1*10000)
ax.set_xlabel('time [s]')
ax.set_ylabel('Damped oscillation [V]')
plt.show()
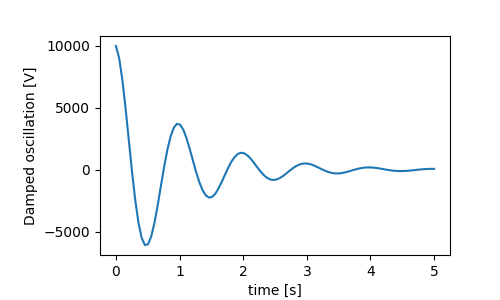
如果要移动标签,可以指定labelpad关键字参数,其中值是点(1/72",与指定字体大小相同的单位)。
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.15, left=0.2)
ax.plot(x1, y1*10000)
ax.set_xlabel('time [s]')
ax.set_ylabel('Damped oscillation [V]', labelpad=18)
plt.show()
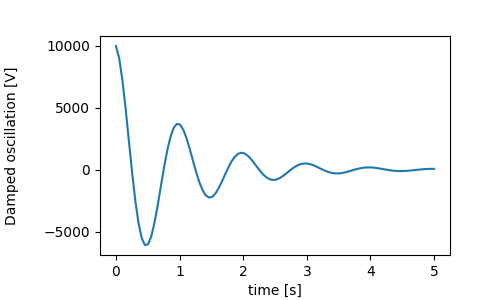
或者,标签接受所有Text
关键字参数,包括
position,我们可以通过它手动指定标签位置。在这里,我们将 xlabel 放在轴的最左侧。请注意,该位置的 y 坐标没有影响 - 要调整 y 位置,我们需要使用
labelpad关键字参数。
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.15, left=0.2)
ax.plot(x1, y1)
ax.set_xlabel('time [s]', position=(0., 1e6), horizontalalignment='left')
ax.set_ylabel('Damped oscillation [V]')
plt.show()
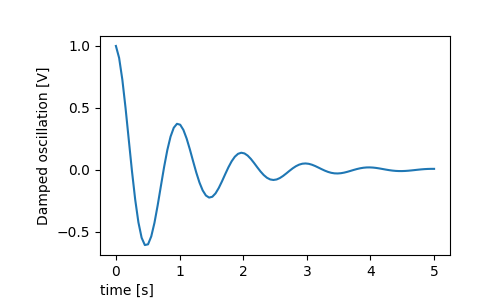
本教程中的所有标签都可以通过操作
matplotlib.font_manager.FontProperties
方法或命名关键字参数来更改set_xlabel
from matplotlib.font_manager import FontProperties
font = FontProperties()
font.set_family('serif')
font.set_name('Times New Roman')
font.set_style('italic')
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.15, left=0.2)
ax.plot(x1, y1)
ax.set_xlabel('time [s]', fontsize='large', fontweight='bold')
ax.set_ylabel('Damped oscillation [V]', fontproperties=font)
plt.show()
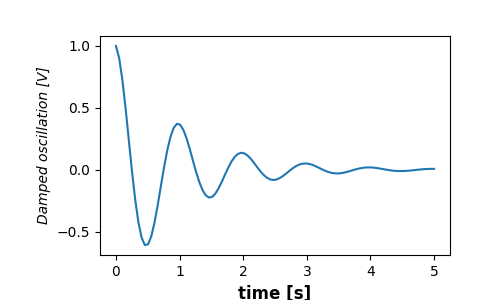
最后,我们可以在所有文本对象中使用本机 TeX 渲染并拥有多行:
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(bottom=0.2, left=0.2)
ax.plot(x1, np.cumsum(y1**2))
ax.set_xlabel('time [s] \n This was a long experiment')
ax.set_ylabel(r'$\int\ Y^2\ dt\ \ [V^2 s]$')
plt.show()
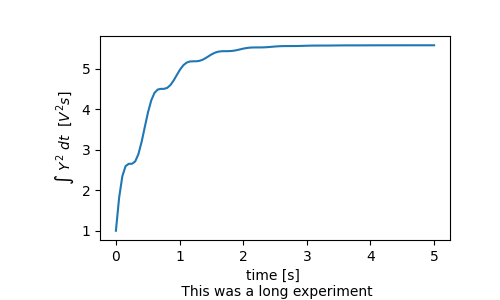
标题#
子图标题的设置方式与标签的设置方式大致相同,但有loc关键字参数可以更改默认值的位置和对齐方式loc=center
。
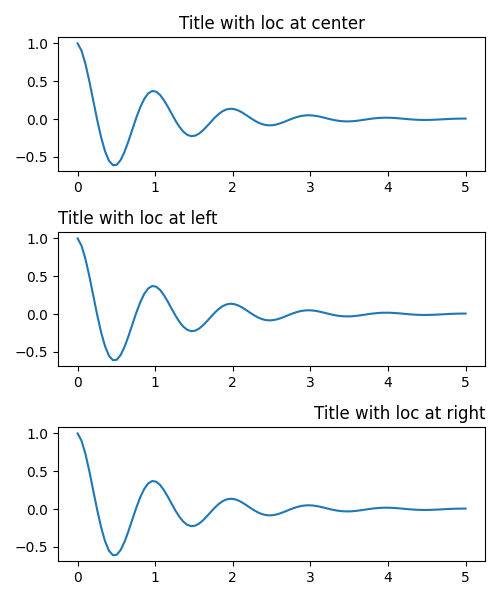
标题的垂直间距通过rcParams["axes.titlepad"]
(默认值:6.0
)控制。设置为不同的值会移动标题。
fig, ax = plt.subplots(figsize=(5, 3))
fig.subplots_adjust(top=0.8)
ax.plot(x1, y1)
ax.set_title('Vertically offset title', pad=30)
plt.show()
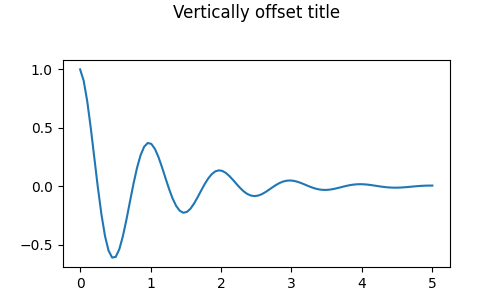
刻度和刻度标签#
放置刻度和刻度标签是制作图形的一个非常棘手的方面。Matplotlib 尽最大努力自动完成任务,但它也提供了一个非常灵活的框架来确定刻度位置的选择以及它们的标记方式。
术语#
轴有一个用于和
的matplotlib.axis.Axis
对象,其中包含有关轴中标签如何布局的信息。ax.xaxis
ax.yaxis
轴 API 在
axis
.
Axis 对象具有主要和次要刻度。Axis 具有
使用绘制的数据确定主要和次要刻度位置的方法Axis.set_major_locator
。Axis.set_minor_locator
还有一些格式化刻度标签Axis.set_major_formatter
的Axis.set_minor_formatter
方法。
简单的记号#
简单地定义刻度值通常很方便,有时也定义刻度标签,覆盖默认的定位器和格式化程序。不鼓励这样做,因为它破坏了绘图的交互式导航。它还可以重置轴限制:请注意,第二个图有我们要求的刻度,包括那些远远超出自动视图限制的刻度。
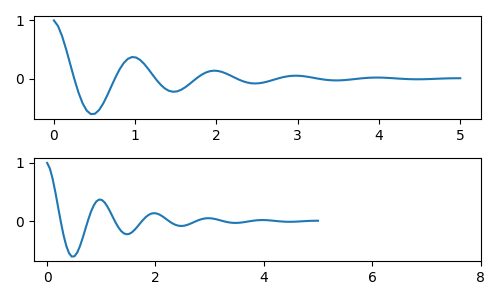
我们当然可以在事后解决这个问题,但它确实突出了硬编码刻度的弱点。此示例还更改了刻度的格式:
fig, axs = plt.subplots(2, 1, figsize=(5, 3), tight_layout=True)
axs[0].plot(x1, y1)
axs[1].plot(x1, y1)
ticks = np.arange(0., 8.1, 2.)
# list comprehension to get all tick labels...
tickla = [f'{tick:1.2f}' for tick in ticks]
axs[1].xaxis.set_ticks(ticks)
axs[1].xaxis.set_ticklabels(tickla)
axs[1].set_xlim(axs[0].get_xlim())
plt.show()
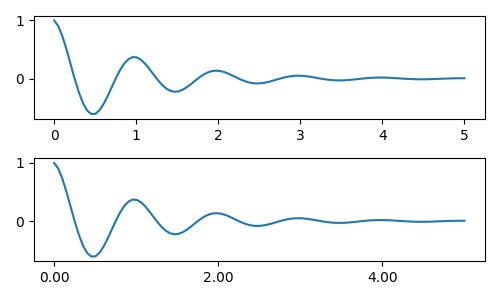
刻度定位器和格式化程序#
我们可以使用matplotlib.ticker.StrMethodFormatter
(new-style str.format()
format string) 或matplotlib.ticker.FormatStrFormatter
(old-style '%' format string) 并将其传递给ax.xaxis
. A
matplotlib.ticker.StrMethodFormatter
也可以通过传递 a 来创建,
str
而无需显式创建格式化程序。
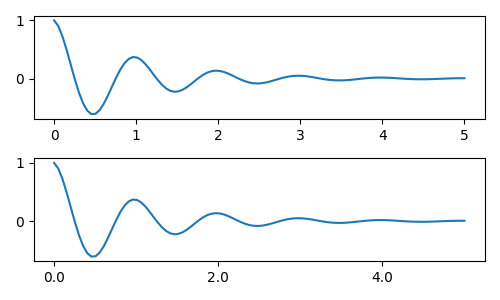
当然,我们可以使用非默认定位器来设置刻度位置。请注意,我们仍然传递刻度值,但不需要上面使用的 x-limit修复。
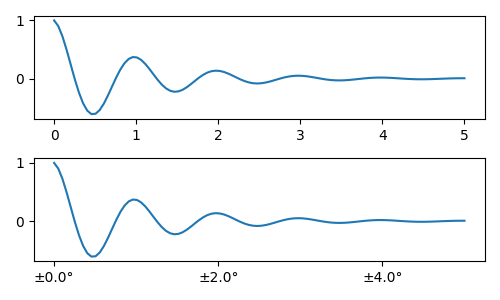
默认格式化程序是被matplotlib.ticker.MaxNLocator
称为
的步骤关键字包含可用于刻度值的倍数列表。即在这种情况下,2、4、6 是可接受的刻度,20、40、60 或 0.2、0.4、0.6 也是如此。但是,3、6、9 是不可接受的,因为 3 没有出现在步骤列表中。ticker.MaxNLocator(self, nbins='auto', steps=[1, 2, 2.5, 5, 10])
nbins=auto
使用算法根据轴的长度确定可接受的刻度数。考虑了刻度标签的字体大小,但不考虑刻度字符串的长度(因为它尚不知道。)在底行,刻度标签非常大,因此我们设置nbins=4
使标签适合右侧-手情节。
fig, axs = plt.subplots(2, 2, figsize=(8, 5), tight_layout=True)
for n, ax in enumerate(axs.flat):
ax.plot(x1*10., y1)
formatter = matplotlib.ticker.FormatStrFormatter('%1.1f')
locator = matplotlib.ticker.MaxNLocator(nbins='auto', steps=[1, 4, 10])
axs[0, 1].xaxis.set_major_locator(locator)
axs[0, 1].xaxis.set_major_formatter(formatter)
formatter = matplotlib.ticker.FormatStrFormatter('%1.5f')
locator = matplotlib.ticker.AutoLocator()
axs[1, 0].xaxis.set_major_formatter(formatter)
axs[1, 0].xaxis.set_major_locator(locator)
formatter = matplotlib.ticker.FormatStrFormatter('%1.5f')
locator = matplotlib.ticker.MaxNLocator(nbins=4)
axs[1, 1].xaxis.set_major_formatter(formatter)
axs[1, 1].xaxis.set_major_locator(locator)
plt.show()
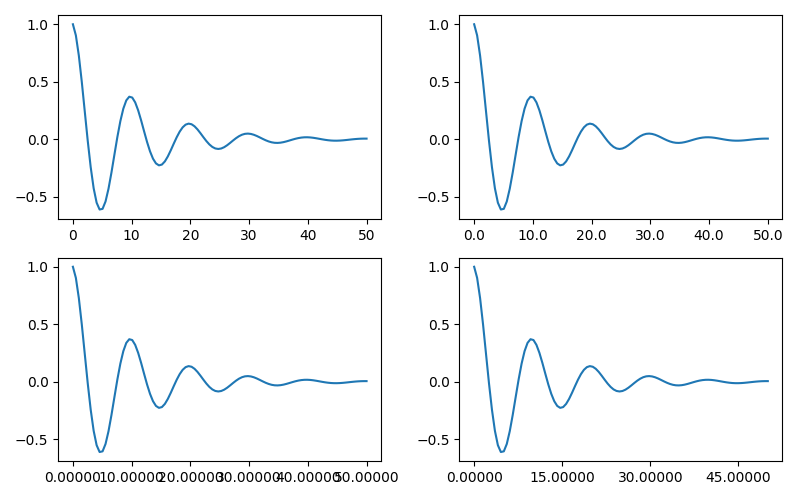
最后,我们可以使用
matplotlib.ticker.FuncFormatter
. 此外,像
matplotlib.ticker.StrMethodFormatter
,传递一个函数将自动创建一个matplotlib.ticker.FuncFormatter
.
def formatoddticks(x, pos):
"""Format odd tick positions."""
if x % 2:
return f'{x:1.2f}'
else:
return ''
fig, ax = plt.subplots(figsize=(5, 3), tight_layout=True)
ax.plot(x1, y1)
locator = matplotlib.ticker.MaxNLocator(nbins=6)
ax.xaxis.set_major_formatter(formatoddticks)
ax.xaxis.set_major_locator(locator)
plt.show()
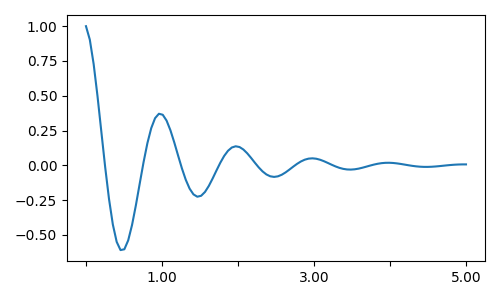
日期标记#
Matplotlib 可以接受datetime.datetime
和numpy.datetime64
对象作为绘图参数。日期和时间需要特殊格式,这通常可以从手动干预中受益。为了提供帮助,日期在matplotlib.dates
模块中定义了特殊的定位器和格式化器。
一个简单的例子如下。请注意我们必须如何旋转刻度标签,以使它们不会相互过度运行。
import datetime
fig, ax = plt.subplots(figsize=(5, 3), tight_layout=True)
base = datetime.datetime(2017, 1, 1, 0, 0, 1)
time = [base + datetime.timedelta(days=x) for x in range(len(x1))]
ax.plot(time, y1)
ax.tick_params(axis='x', rotation=70)
plt.show()
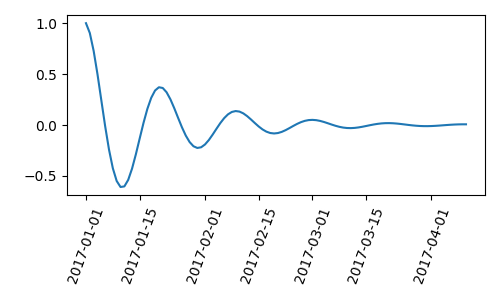
我们可以将格式传递给matplotlib.dates.DateFormatter
. 另请注意,29 日和下个月非常接近。我们可以通过使用dates.DayLocator
类来解决这个问题,它允许我们指定要使用的月份中的日期列表。matplotlib.dates
模块中列出了类似的格式化程序。
import matplotlib.dates as mdates
locator = mdates.DayLocator(bymonthday=[1, 15])
formatter = mdates.DateFormatter('%b %d')
fig, ax = plt.subplots(figsize=(5, 3), tight_layout=True)
ax.xaxis.set_major_locator(locator)
ax.xaxis.set_major_formatter(formatter)
ax.plot(time, y1)
ax.tick_params(axis='x', rotation=70)
plt.show()
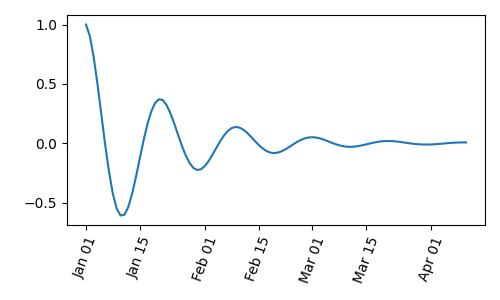
图例和注释#
脚本总运行时间:(0分5.998秒)